Password authentication for web and mobile apps
Authenticating users with passwords is a fundamental part of web and mobile security. It is also the part that's easy to get wrong.
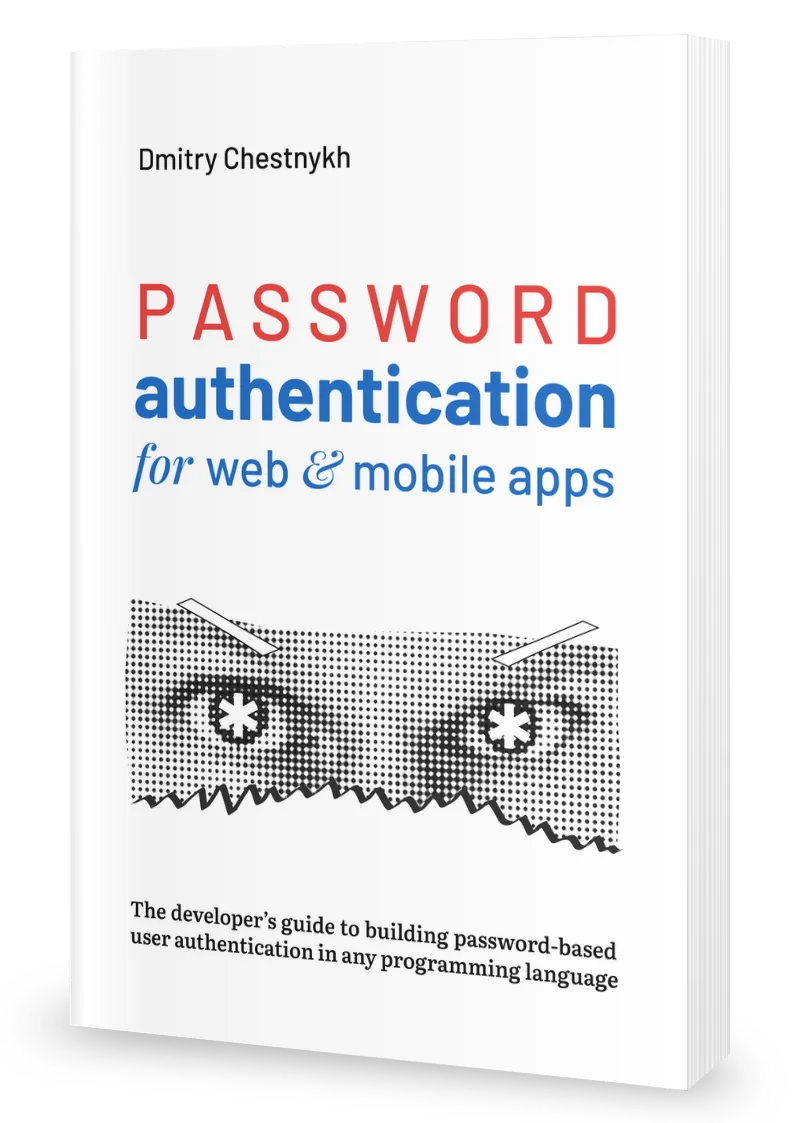
This book is for developers who want to learn how to implement password authentication and log in flows correctly and securely.
It answers many questions that everyone has when writing their own authentication system or learning a framework that implements it.
Store passwords securely
- What is the best password hashing function for your app?
- How many bytes of salt should you use?
- What is the optimal password hash length?
- How to encode and store hashes?
- When to pepper and encrypt hashes and how to do it securely?
- How to avoid vulnerabilities in bcrypt, PBKDF2, and scrypt, and which Argon2 version to use?
- How to update password hashes to keep up with Moore’s law?
- How to enforce password quality?
Remember users
- How to implement secure sessions that are not vulnerable to timing attacks and database leaks?
- Why is it a bad idea to use JWT and signed cookies for sessions?
- How to allow users to view and revoke sessions from other devices?
Verify usernames and email addresses
- How to verify email addresses and why is it important? How Skype failed to do it and got hacked.
- How to avoid vulnerabilities caused by Unicode?
- How to disallow profanities and reserved words in usernames?
Add multi-factor authentication
- How to implement two-factor authentication with TOTP and WebAuthn/U2F security keys?
- How to generate recovery codes? How long should they be?
- How to rate limit 2FA and why not doing it breaks everything?
Also…
- How to create accessible registration and log in forms?
- How to use cryptography to improve security? When to avoid it?
- How to generate random strings that are free from modulo bias?
The book applies to any programming language: it explains concepts and algorithms in English and provides references to relevant libraries for popular programming languages.
Contents
- Introduction
Basics
- Cryptography
- Randomness
- Modulo bias
- UUID
- Constant-time comparison
- CSRF protection
- Audit logging and reporting
Users
- Usernames vs email addresses
- Filtering usernames
- Validating email addresses
- Confirming email addresses
- Universal confirmation system
- Changing usernames
- Changing email addresses
- Requiring re-authentication
- Unicode issues
Passwords
- Password quality control
- Characters and emoji in passwords
- Password hashing
- PBKDF2
- bcrypt
- scrypt
- yescrypt
- Argon2
- Goodbye, rainbow tables
- Prehashing for scrypt and bcrypt
- Peppering and encrypting hashes
- Hash length
- Encoding and storing password hashes
- Hash upgrades
- Client-side password prehashing
- Resetting passwords
- Changing passwords
- Against enforced password rotation
- Secret questions
Multi-factor authentication
- What is multi-factor authentication?
- Two-step or two-factor?
- Rate limiting
- TOTP
- SMS
- Security keys: U2F, WebAuthn
- Registration
- Authentication
- Resources
- Recovery codes
Sessions
- JWT and signed cookies for sessions?
- Client-side session data
- Signed cookies
- Encrypted cookies
- Sessions list
- Revoking sessions
- Logging out
- Server-side storage of sessions
- Client-side storage of session tokens
- Web apps
- Mobile apps
Usability and accessibility
- Registration form
- Sign in form
- Afterword
- About the author
- Index
Buy e-book directly from me
Get Password authentication for web and mobile apps for the introductory price before I raise it!
130-page e-book
Formats included (all DRM-free):
- ePub (PC, Mac, iPhone, iPad, Android)
- mobi (Kindle)
Buy paperback or e-book from retailers
Paperback or Kindle
Amazon, Rakuten Kobo, and Barnes & Noble are trademarks of their respective companies.
Some Amazon links are affiliate links. Cats were involved in the production of this book.
Some Amazon links are affiliate links. Cats were involved in the production of this book.